Learn to test iOS in-app subscriptions effectively with our guide on setup, automation, and troubleshooting.

Introduction
Integrating subscription services in mobile apps is a great way for businesses to earn steady income and keep users engaged. Subscriptions let users pay regularly for access to services or content, which helps businesses predict earnings and build a loyal user base. This approach is widely used in areas like media streaming and productivity apps where continuous user interaction is key.
However, setting up and managing these subscriptions can be complex, especially on iOS devices. Apple provides powerful tools and strict guidelines for subscriptions, which require careful testing to ensure everything works as it should. Proper testing helps avoid issues like payment errors or service interruptions, which can frustrate users and impact a business’s revenue. Ensuring these systems work smoothly is crucial for maintaining a reliable service that users trust.
Understanding In-App Subscriptions on iOS
In-app subscriptions are a mechanism within iOS apps that allows users to purchase access to content or services on a recurring basis. This recurring revenue model is integral to many modern business strategies, especially in apps related to media, productivity, or lifestyle services.
iOS primarily supports two types of subscriptions:
- Auto-renewable Subscriptions: These are the most common type and automatically renew at the end of each subscription period until the user decides to cancel. This model is ideal for services requiring ongoing access, like streaming platforms or continuous software updates.
- Non-renewing Subscriptions: Unlike auto-renewable, these do not automatically renew and must be repurchased periodically by the user. They are suitable for temporary events or seasonal content, such as a sports season pass.
Each type has distinct management practices and testing requirements which are crucial for ensuring a smooth user experience and compliance with Apple’s guidelines.
Key Components of iOS Subscriptions
To effectively integrate and manage in-app subscriptions, understanding the key components involved is crucial. These include:
- StoreKit Framework: This is the core of in-app purchases and subscriptions on iOS. It handles everything from displaying products to processing payments.
- Product ID: Each subscription type is identified by a unique product ID, which is essential in tracking and managing subscriptions within the app.
- Receipt Validation: To prevent fraud and ensure all transactions are legitimate, iOS uses receipt validation. This confirms that the purchase was made through the App Store and not altered in any way.
- Subscription Groups: Useful for managing user upgrades or downgrades between different subscription options, allowing users to switch between various service levels without multiple charges.
Understanding these components is vital for developing a strong testing strategy. Testing not only ensures that subscriptions are handled correctly within the app but also verifies compliance with Apple’s payment and subscription guidelines, which are stringent to protect users from unintentional subscriptions and to provide clear information about the subscription terms.
Setting Up the Test Environment
Requirements for Setting Up a Sandbox Environment
Apple provides a Sandbox testing environment specifically for testing in-app purchases, including subscriptions. This environment allows you to test purchases without incurring actual charges. To set up a Sandbox environment, you'll need:
- An active Apple Developer account.
- A test Apple ID created within your Apple Developer account to simulate the user experience without affecting a real user's account status or billing.
Configuring Apple Developer Account for Testing
To configure your Apple Developer account for testing in-app subscriptions:
- Register your app: Ensure your app is registered in the Apple Developer portal with a unique Bundle ID.
- Create Sandbox Testers: In the Users and Access section, add new Sandbox tester accounts. These accounts mimic real user accounts and can be used to verify the complete subscription lifecycle.
- Define Products: Set up your in-app purchases in App Store Connect by specifying product IDs, pricing, and descriptions for each type of subscription you offer.
Creating Test Users
Creating test users is straightforward but crucial for a thorough test cycle. Follow these steps:
- Add Test Users: Within App Store Connect, navigate to 'Users and Roles', then 'Sandbox Testers'. Click 'Plus' to add a new tester.
- Provide Details: Enter the details for the test users, including an email address and password. Ensure these details are different from your actual Apple ID to avoid conflicts.
- Verify Emails: Each test user will receive an email to verify their account, which is necessary to activate the sandbox testing capability.
Code Snippet: Setting Up Sandbox Testers
To automate part of this setup, you can use scripts or management tools. Here’s a basic Python script example that outlines how you might manage Sandbox testers programmatically (note: actual implementation will depend on your backend and security requirements):
Python
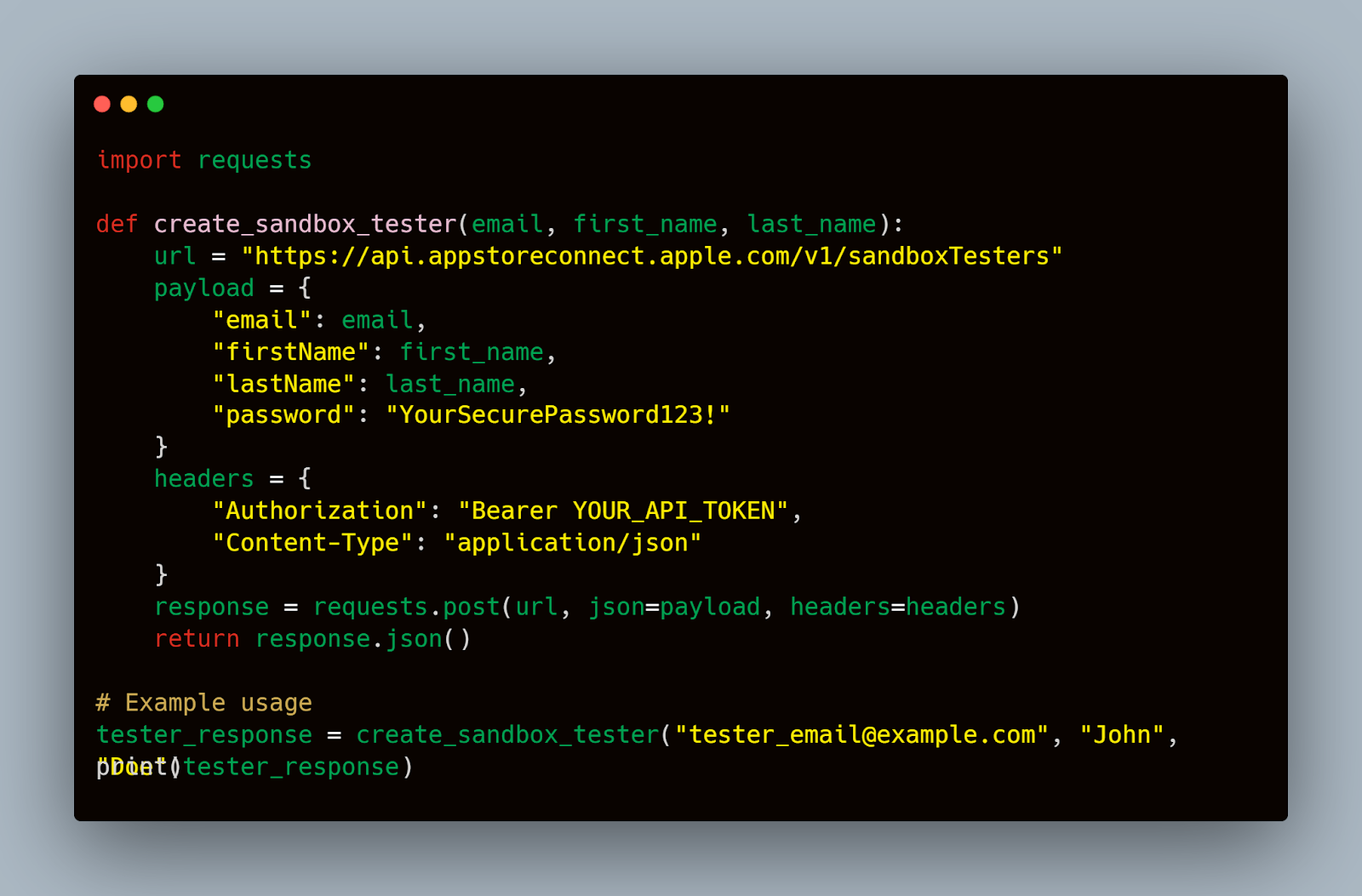
This script demonstrates how to programmatically add a sandbox tester to your Apple Developer account using the App Store Connect API. Be sure to replace placeholders with actual data and secure handling mechanisms as required.
Setting up a comprehensive test environment is pivotal for uncovering potential issues in subscription management before they impact users. By following these guidelines, you can ensure that your testing is thorough, reflecting real-world usage as closely as possible. This preparatory work is essential for the smooth deployment and operation of subscription-based services in your iOS app.
Implementing In-App Purchases in Your App
Code Integration Using StoreKit
StoreKit is the framework provided by Apple that enables the incorporation of in-app purchases into your iOS applications. It handles the communication with the Apple App Store to manage purchases, verify receipts, and maintain subscriptions. Here's a step-by-step guide to get started:
- Import StoreKit: Start by importing the StoreKit framework into your iOS project to access its functionalities.
Swift
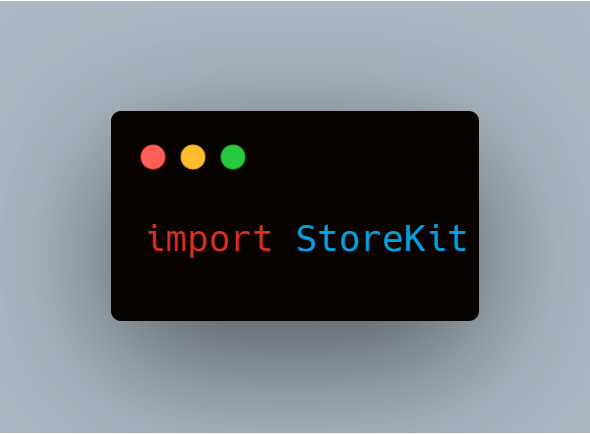
2. Request Products: Your app needs to request the product information from the App Store based on the product IDs you've set up in your Apple Developer account.
Swift
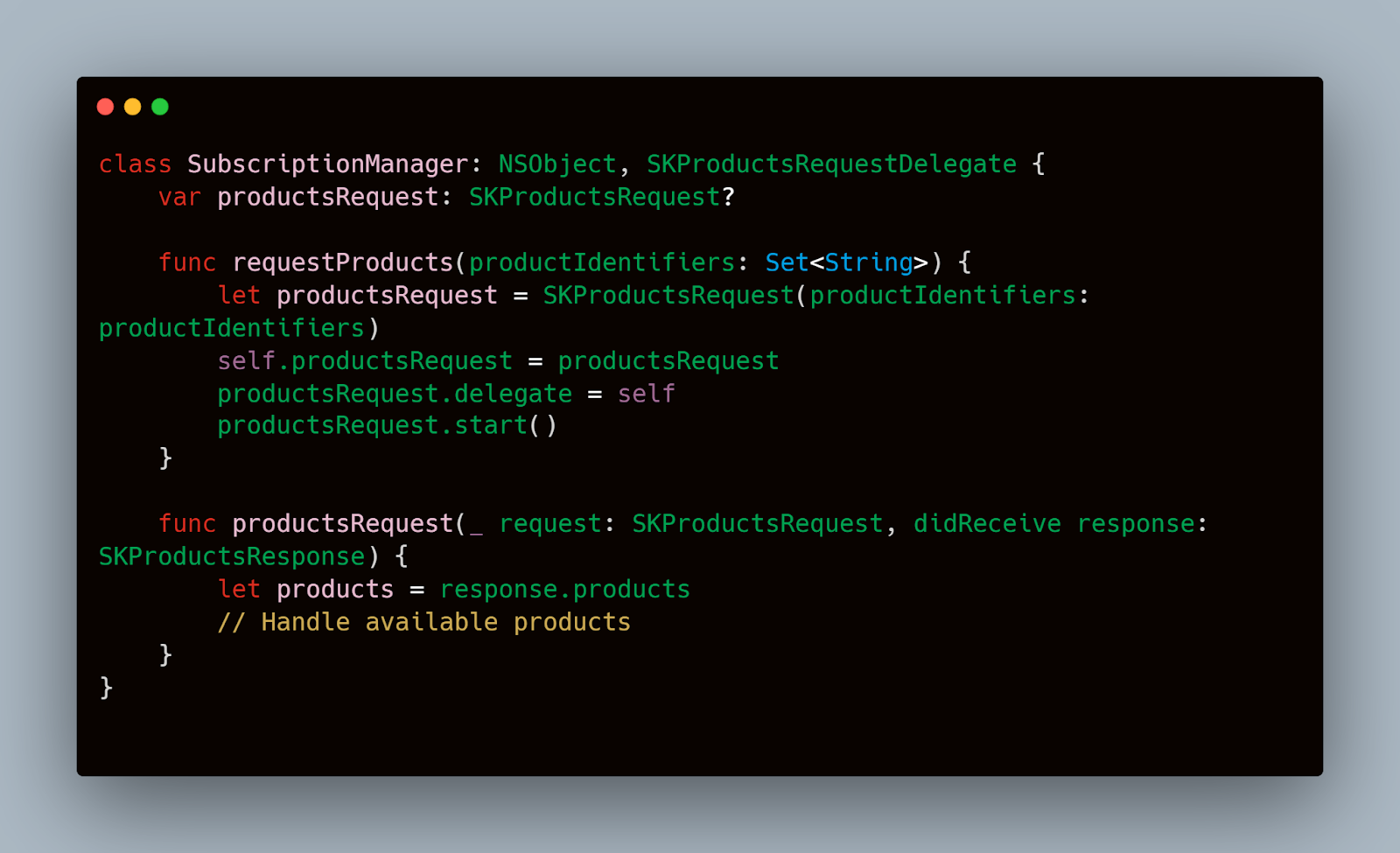
3. Initiate Purchase: Once the products are fetched, you can present them to the user and handle the purchase request.
Swift
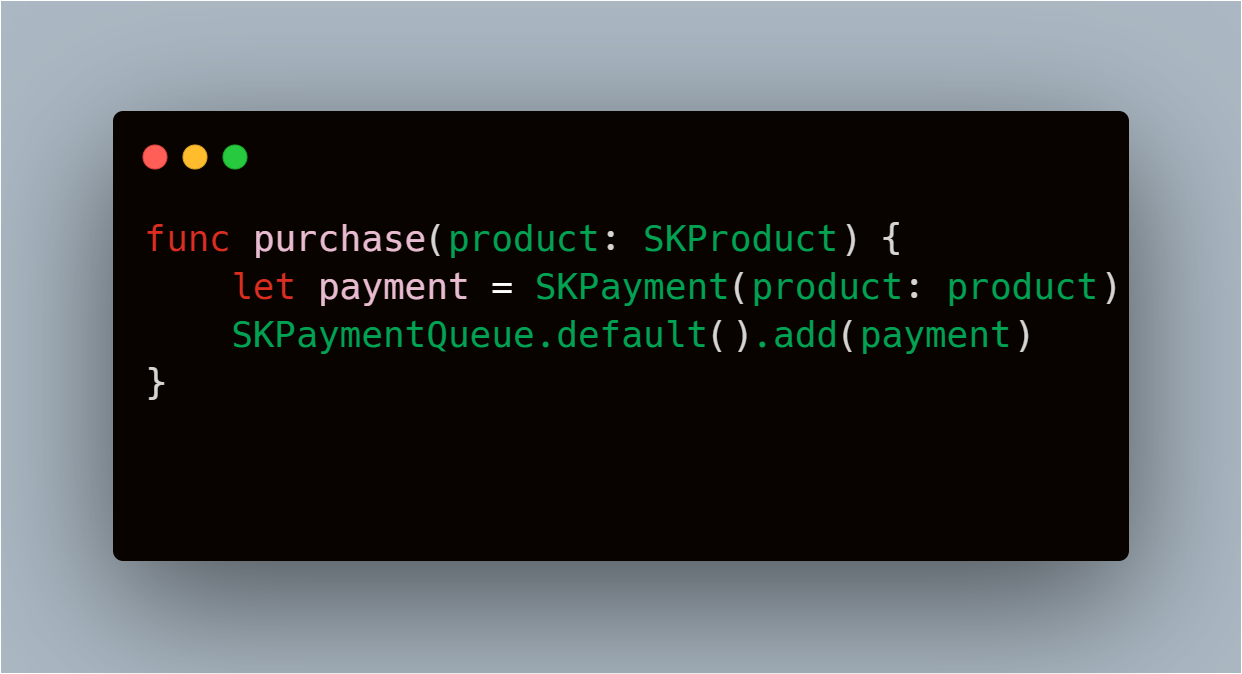
4. Transaction Handling: Monitor and handle transaction updates by observing the payment queue.
Swift

Handling Purchase Flow in the App
Managing the user's purchase flow involves not only initiating and processing purchases but also ensuring a good user experience by handling errors and providing appropriate feedback.
- User Interface: Provide a clear and informative interface for the user to select subscription options and understand what they are purchasing.
- Error Handling: Implement strong error handling to manage scenarios where transactions fail due to network issues, cancellations, or other errors.
Swift

Integrating and managing in-app subscriptions meticulously not only complies with Apple’s guidelines but also enhances user trust by ensuring transactions are smooth and transparent. By following these steps and utilizing the provided code snippets, developers can effectively implement a strong subscription system within their iOS apps.
Testing Scenarios
Initial Purchase Tests
The initial purchase is the first point of interaction with the subscription service for many users, making it crucial to test for a seamless and error-free experience. Here are specific scenarios to consider:
- Successful Purchase: Validate that the subscription is correctly activated upon a successful payment. Ensure that the user’s account reflects the new subscription status immediately.
- Payment Decline: Simulate declined payments to verify that the app handles these gracefully, informing the user of the issue without compromising the user experience.
- Expired Payment Information: Test with outdated payment information to check if the app prompts the user to update their payment details.
Swift

Renewal Tests
Subscription renewals are as significant as initial purchases because they reflect the ongoing user commitment. Test these scenarios to ensure subscriptions renew as intended:
- Automatic Renewal: Ensure that the app correctly processes a subscription renewal at the end of the billing period.
- Cancellation Handling: Confirm that when a subscription is canceled, the service discontinues appropriately after the current billing period ends.
Edge Cases
Edge cases can significantly affect the user experience if not handled correctly. Here are a few to consider:
- Network Failures: Test the app’s response to network interruptions during a transaction to ensure it can recover gracefully without losing user data or duplicating transactions.
- Simultaneous Logins: Verify that the subscription access is consistent across multiple devices logged in with the same user account.
Swift

This detailed approach not only aids in uncovering functional flaws but also enhances the overall quality of the subscription service offered in your iOS application.
Using Tools and Services to Automate Testing
Several tools are available to automate UI testing for iOS apps, each with its strengths. Some popular options include:
- Mobot: A service that automates mobile testing using robots and real devices. It's particularly useful for routine tasks and regression tests, ensuring that your app’s subscription functionalities work correctly across different device configurations.
- XCTest: Apple's native framework that integrates seamlessly with Xcode, providing a strong suite of functionalities for UI testing.
- Appium: An open-source tool that allows for cross-platform testing, which means you can use the same test scripts for both iOS and Android.
Setting Up Mobot for Recurring Subscription Tests
Using Mobot to automate testing of recurring subscriptions involves a few specific steps:
- Define Test Cases: Clearly outline what each test should cover, such as successful subscription sign-ups, renewals, and handling of failed payments.
- Integrate with Your CI/CD Pipeline: Connect Mobot’s testing services with your continuous integration and delivery pipeline to trigger tests automatically after each build or periodically.
- Analyze Results: Mobot provides detailed logs and video recordings of each test session. Review these results to identify any issues that occur during the subscription process.
Here’s a conceptual example of how you might set up a test for a subscription renewal using Mobot:
Python

This simple script demonstrates a test for checking the renewal functionality of a subscription within an iOS app using Mobot. It ensures that the app correctly processes a renewal, which is critical for maintaining user subscriptions without interruption.
Benefits of Automated Testing
Automated testing, especially when integrated into development workflows, offers numerous benefits:
- Consistency: Tests are performed the same way every time, eliminating variability in results due to different testing approaches or human error.
- Efficiency: Automated tests can run frequently and quickly, allowing for more rapid iterations and faster feedback on changes.
- Coverage: Automation makes it feasible to run comprehensive tests covering all features and edge cases, which might be impractical manually.
Incorporating tools like Mobot into your testing strategy can significantly improve the efficiency and effectiveness of your QA processes, ensuring that your in-app subscription features provide a seamless experience for users across all scenarios.
Analyzing Test Results
Interpreting Logs from Sandbox Tests
When conducting tests in a sandbox environment, detailed logs are generated that record every transaction and system interaction. These logs are vital for tracing the flow of transactions and identifying where failures or unexpected behaviors occur.
- Success Indicators: Look for confirmation codes and success statuses that indicate a transaction has been processed correctly.
- Error Logs: Analyze logs for error messages or failure codes. Determine if these are caused by bugs in the app, issues with sandbox setup, or external factors like network problems.
For example, a typical log entry after a successful subscription purchase might look like this:
Plaintext
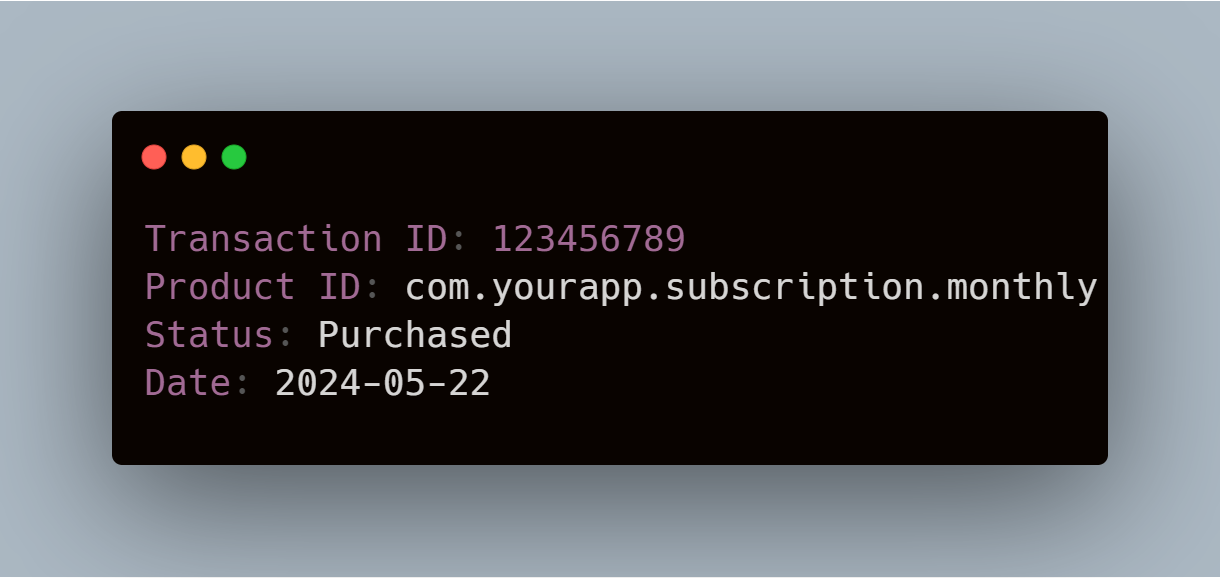
And for a failed transaction:
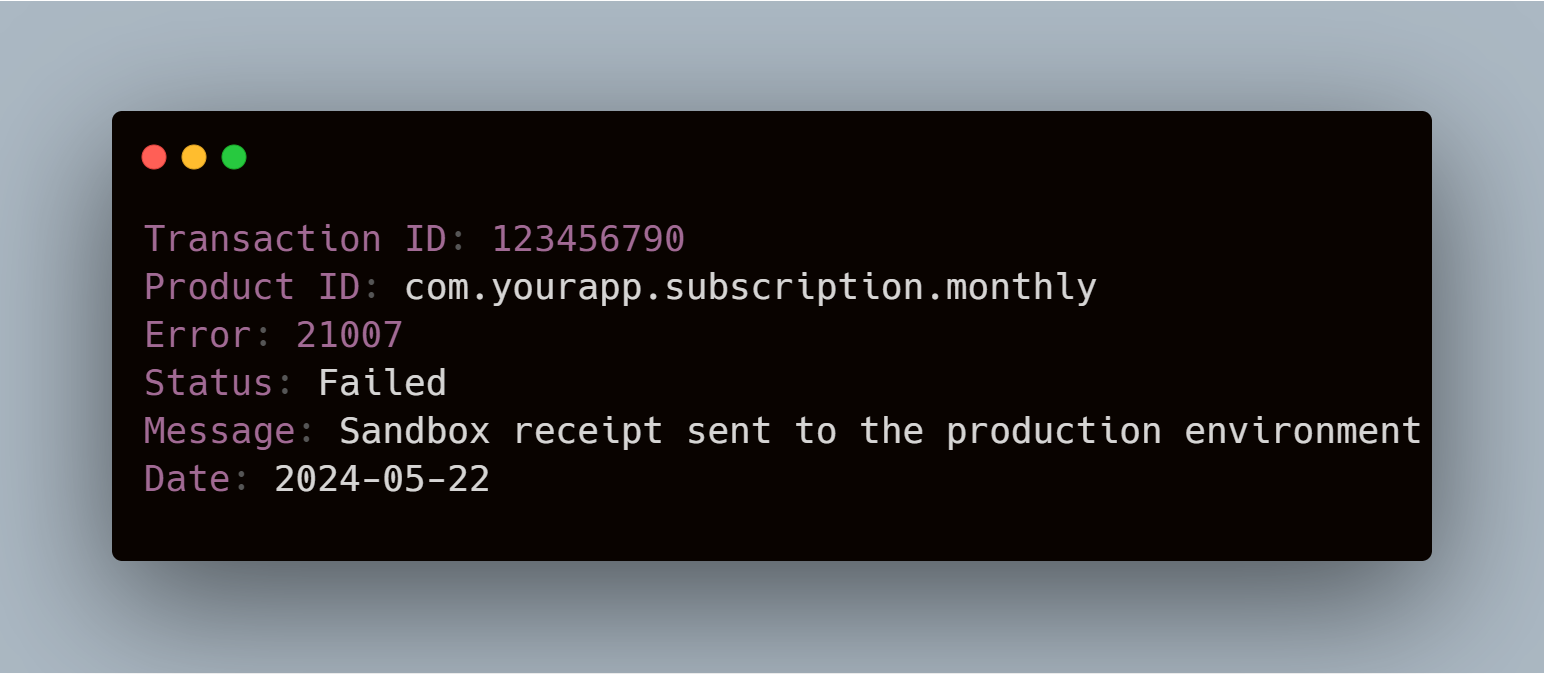
This error indicates a common mistake where a sandbox receipt is mistakenly sent to the production payment gateway.
Important Metrics to Monitor
Several key metrics can provide insights into the performance and reliability of your subscription services:
- Transaction Success Rate: The percentage of successful transactions compared to total transactions. A low success rate might indicate issues in the purchase flow or with the payment gateway.
- Renewal Rate: Measures the percentage of subscriptions that are successfully renewed. It helps assess the long-term viability of your subscription model.
- Failure Reasons: Categorizing and quantifying the reasons for transaction failures can guide targeted improvements.
Using Visualization Tools
Utilizing data visualization tools can help in understanding complex data sets and identifying trends or anomalies over time. Tools like Grafana or Tableau can create intuitive dashboards that display real-time data from your tests.
Swift

Continuous Improvement
The ultimate goal of analyzing test results is not just to identify problems but to foster a cycle of continuous improvement. Insights derived from test results should lead to actionable steps that enhance system performance, streamline user experience, and increase overall subscription success rates.
By systematically analyzing test results and employing data effectively, teams can ensure that their app's subscription functionalities are not only efficient but also aligned with user expectations and business objectives. This analysis is essential for maintaining a competitive edge and achieving long-term success in the app marketplace.
Troubleshooting Common Issues
1. Identifying Common Pitfalls
During the lifecycle of in-app subscription testing, several recurring issues may arise:
- Authentication Errors: Issues where users cannot authenticate or login to access their subscriptions.
- Payment Failures: Transactions that fail due to issues with payment methods or network connectivity.
- Subscription Sync Errors: Problems with syncing subscription status across multiple devices.
2. Strategies for Diagnosing Issues
To effectively address these issues, employ a systematic approach to diagnosis:
- Log Analysis: Review application and system logs to trace the source of errors. Look for error codes or messages that can pinpoint the exact problem.
- Simulating User Scenarios: Replicate the user’s actions that led to the issue. This approach helps in understanding the context of the problem.
- Network Monitoring: Use tools to monitor network requests and responses. This can be crucial for identifying issues related to payment processing or data synchronization.
Consider a scenario where a user reports a failed subscription renewal. Here’s how you might approach troubleshooting this issue:
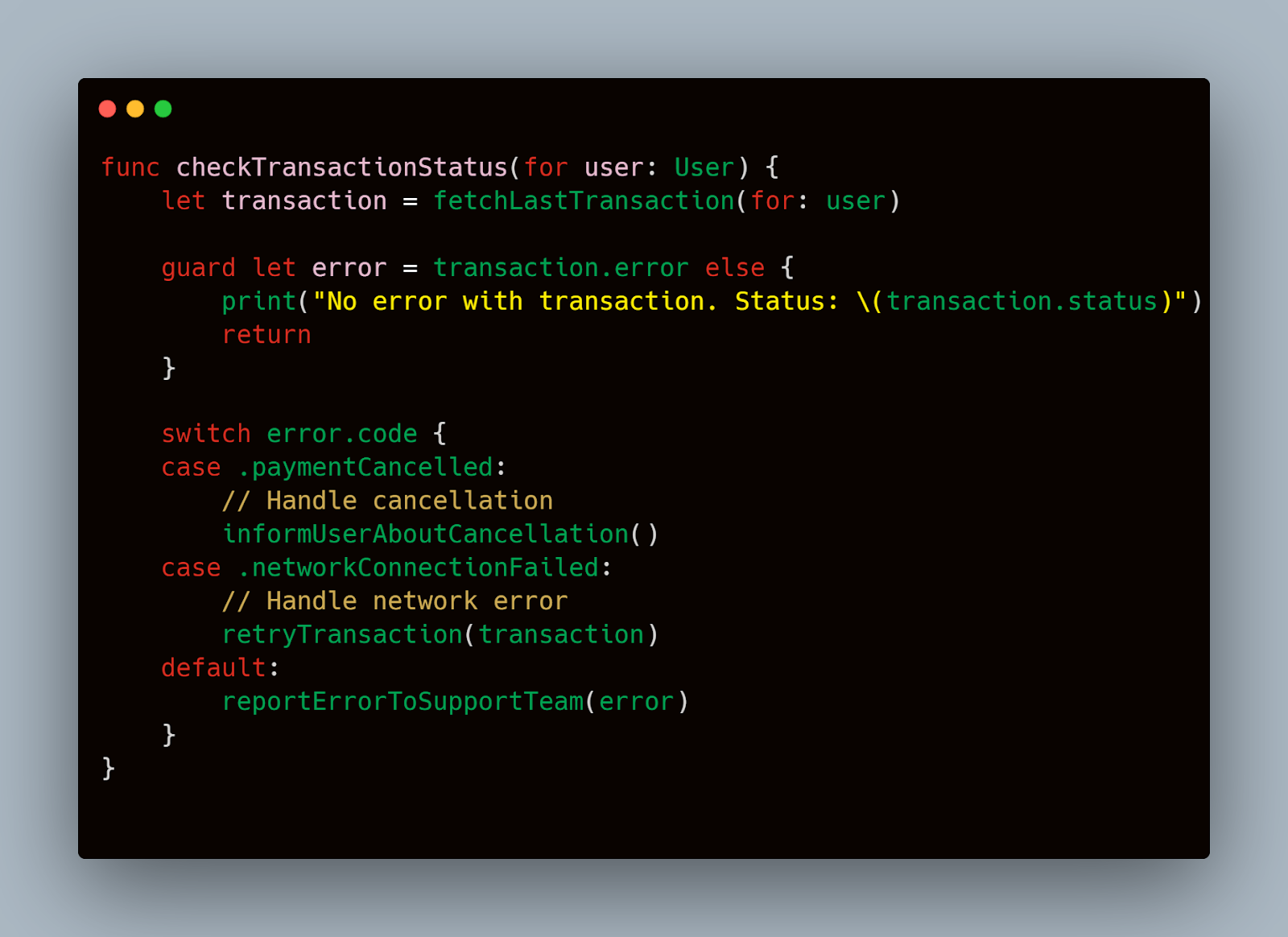
This snippet demonstrates how to diagnose a transaction failure based on error codes, providing targeted solutions based on the specific issue.
3. Common Fixes and Workarounds
Once issues are identified and diagnosed, apply appropriate fixes:
- Updating Payment Information: If payment failures are frequent, ensure the app prompts users to check and update their payment details.
- Improving Error Messages: Provide clear and informative error messages to guide users when something goes wrong.
- Enhancing Network Resilience: Implement retry mechanisms and strengthen error handling to improve the app’s resilience to network issues.
4. Preventive Measures
Prevention is better than cure. To minimize the occurrence of these issues:
- Comprehensive Testing: Regularly test all aspects of the subscription process under various conditions and user scenarios.
- Feedback Loops: Encourage user feedback and rapidly incorporate this into your troubleshooting and development processes.
- Continuous Monitoring: Use monitoring tools to keep an eye on system performance and quickly detect and address new issues as they arise.
By employing these troubleshooting techniques and preventive strategies, teams can significantly reduce the incidence and impact of common subscription-related issues, ensuring a smooth and reliable experience for all users. This proactive approach not only addresses problems more efficiently but also builds user trust and satisfaction with your subscription services.
Conclusion
By thoroughly understanding the integration and operation of subscriptions, setting up detailed test environments, automating repetitive tasks, and proficiently handling common issues, teams can significantly enhance the reliability and user experience of their apps. Moreover, diligent analysis of test results and proactive troubleshooting ensure continuous improvement, keeping your app compliant with Apple’s standards and expectations. Adopting these best practices will not only improve the quality of your service but also fortify your app’s reputation in the competitive app marketplace, ultimately driving user satisfaction and retention.