Learn essential strategies for testing iOS app background behavior to ensure reliability and efficiency.
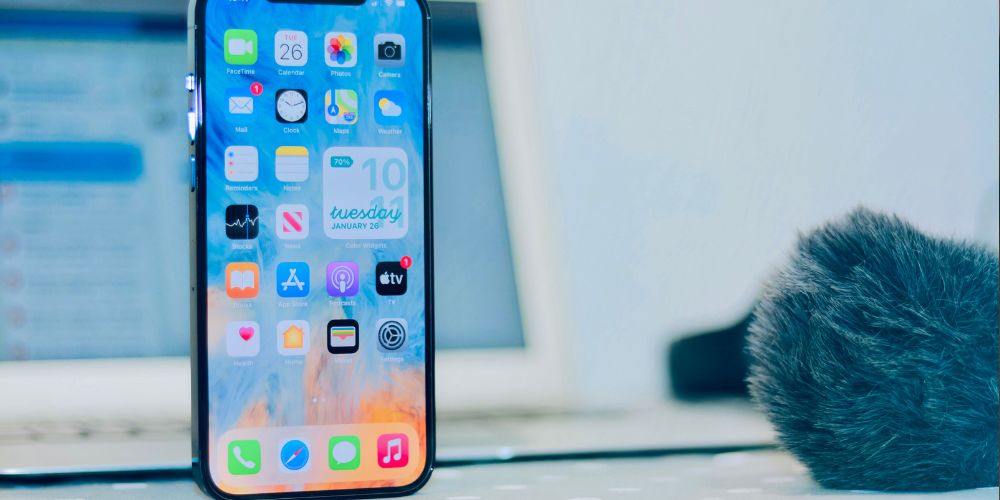
Introduction
When developing mobile applications, especially for iOS, backgrounding—how an app behaves when it's not in the foreground—is an aspect that cannot be overlooked. Testing how an app transitions to and from the background is crucial for ensuring it remains stable and functional, irrespective of the user's interaction pattern. This isn't just about preventing the app from crashing; it's about delivering a seamless user experience that conserves battery life and system resources, while still performing necessary tasks effectively.
iOS devices uniquely handle background operations, designed to optimize user experience and manage system resources. From playing audio to updating locations, iOS allows apps limited functionality while running in the background. This presents a distinct challenge: ensuring that their app behaves as expected, even when not directly visible to the user.
Effective background testing covers a range of scenarios, from simple network requests to complex interactions with system APIs. It ensures that when users switch from your app to answer a call or reply to a text, they return to your app with their progress and data intact. More importantly, if your app is set to perform tasks in the background, such as downloading content or refreshing data, these operations must be executed correctly without unnecessary drain on device resources.
The consequences of inadequate background testing can be significant. Users might face data loss, or apps might consume excessive battery power, leading to poor reviews and reduced user retention. In more severe cases, improper handling of background processes could lead to security vulnerabilities, especially when dealing with sensitive user data.
Understanding Background Modes in iOS
In iOS, managing how an application behaves when not in the foreground is crucial to ensuring user satisfaction and optimal device performance. iOS supports several background modes, each designed for specific types of applications and functionalities. These include:
- Audio and AirPlay: Allows apps to continue playing audio or stream audio to AirPlay devices even when the app is not in the foreground.
- Location updates: Enables apps to receive updates about changes in the user’s location, crucial for navigation or fitness-tracking applications.
- Background fetch: Periodically wakes the app in the background to fetch updates or new content in preparation for the next user interaction.
- Remote notifications: Allows the app to be awakened in response to a push notification.
- Background Bluetooth: Supports apps that need to communicate with Bluetooth devices in the background.
Each of these modes requires specific settings in both the app’s Info.plist file and within the app’s code to declare and handle the background tasks appropriately.
Configuring Your App for Background Execution
To enable background modes, you must first declare the types of background modes your app intends to use in the Info.plist file. This is done by adding the UIBackgroundModes key and specifying the appropriate values. For example, if your app needs to perform background fetches and play audio, your Info.plist configuration would look something like this:
Xml

Once the modes are declared, it’s crucial to implement the application delegate’s methods to manage background tasks efficiently. For example, to handle background fetches, you would implement the application:performFetchWithCompletionHandler: method in your app delegate:
Swift

This method is invoked by iOS when it is an appropriate time to perform a fetch. The completion handler must be called as soon as you're done fetching data to let the system know of your success or failure and to allow the system to estimate your app’s power usage accurately.
Practical Considerations
When configuring your app for background execution, consider the following:
- Minimize power consumption: Only perform necessary activities and defer optional tasks until the app is active.
- Manage task execution time: iOS typically allows apps to run in the background for a short period. Ensure your tasks complete within the time constraints.
- Handle task completion: Always signal the end of a task by calling the appropriate completion handler.
By understanding and implementing these background modes, developers can ensure that their iOS applications perform efficiently and reliably, providing a seamless user experience even when running in the background.
Setting Up the Test Environment
Testing the background capabilities of an iOS app requires a meticulous setup that includes both software and hardware configurations.
Tools and Frameworks Required
To start, you will need the latest version of Xcode, which includes all necessary tools and frameworks for iOS development and testing. It is imperative to keep your development environment up to date to take advantage of the latest features and testing capabilities. You can download it from the Apple Developer website.
Alongside Xcode, using real devices for testing is crucial. While simulators are excellent for the early stages of development due to their convenience and accessibility, they do not mimic the hardware limitations and exact behaviors of actual iOS devices. Therefore, having a range of iOS devices with different configurations and operating system versions is beneficial to cover more scenarios and catch potential issues that could affect a segment of your user base.
Configuring Xcode for Background Testing
Here’s how to set up Xcode to test background behaviors effectively:
- Enable Background Modes: First, ensure your app is configured to use background modes. Go to your project settings in Xcode, select your target, and then navigate to the ‘Capabilities’ tab. Here, you must turn on ‘Background Modes’ and select the appropriate modes that your app will use, as discussed in the previous section.
- Provisioning Profiles and Device Setup: Make sure you have the correct provisioning profiles that allow background tasks. Provisioning profiles need to be properly configured in the Apple Developer account to include the necessary capabilities.
- Network Configuration: If your tests involve networking during background execution (like downloading data or sending notifications), configure your network in Xcode’s scheme settings. Go to Product > Scheme > Edit Scheme, select ‘Run’ from the side menu, and then go to the ‘Arguments’ tab where you can set up environment variables and other runtime configurations.
Using XCTest to Simulate Background Conditions
XCTest framework provides capabilities to simulate and automate tests involving backgrounding. Here is an example of how you can use XCTest to simulate a background transition:
Swift

This test automates the process of sending the app to the background and then bringing it back to the foreground, checking if the app returns to its expected state.
Monitoring Tools
To ensure comprehensive testing, employ monitoring tools like Instruments in Xcode, which can track memory usage, CPU load, and other critical metrics during background execution. This helps in identifying performance issues that could lead to crashes or excessive battery usage.
Automating Background State Transitions
Automating the testing of background state transitions is an essential part of ensuring that iOS apps behave reliably when they move between the foreground and background. This not only helps in verifying that the app saves its state correctly but also ensures that all background tasks are handled efficiently without draining system resources unnecessarily.
Utilizing XCTest for State Transition Automation
XCTest provides a strong framework for automating UI tests, including simulating the app moving to the background and returning to the foreground. This can be crucial for testing how apps handle network communications, data fetching, and other critical operations during state transitions.
Here is an example of how to use XCTest to automate testing of background state transitions:
Swift

This test ensures that your app transitions correctly to the background and then back to the foreground, verifying the app’s state at each step.
Code Snippets for Automating State Changes
To further automate testing, consider implementing background tasks in your test cases. For instance, if your app needs to download data periodically, you can simulate this task using XCTest:
Swift

Integrating Third-Party Services for Enhanced Testing
While XCTest offers extensive tools for automating tests, integrating third-party services can provide additional benefits. For example, Mobot offers automated mobile testing services that can help streamline the testing of background tasks. By employing real mobile devices and sophisticated scripting options, Mobot can simulate complex user interactions and background activities, providing a more comprehensive testing outcome than simulators alone.
Testing Network Calls During Background Execution
Ensuring that an iOS app can handle network calls effectively while in the background is a critical aspect of mobile application development.
Handling API Calls in Background Mode
iOS apps typically have constraints when running in the background, particularly concerning network requests. However, certain apps need to continue making network calls, for instance, to fetch fresh data or send updates to a server. Here’s how you can manage and test these network calls:
- Using Background Tasks for Network Operations: To initiate network calls in the background, iOS developers can use URLSession configured with a background session configuration. This setup allows the app to perform network transfers even when the app is suspended or not running.
Swift
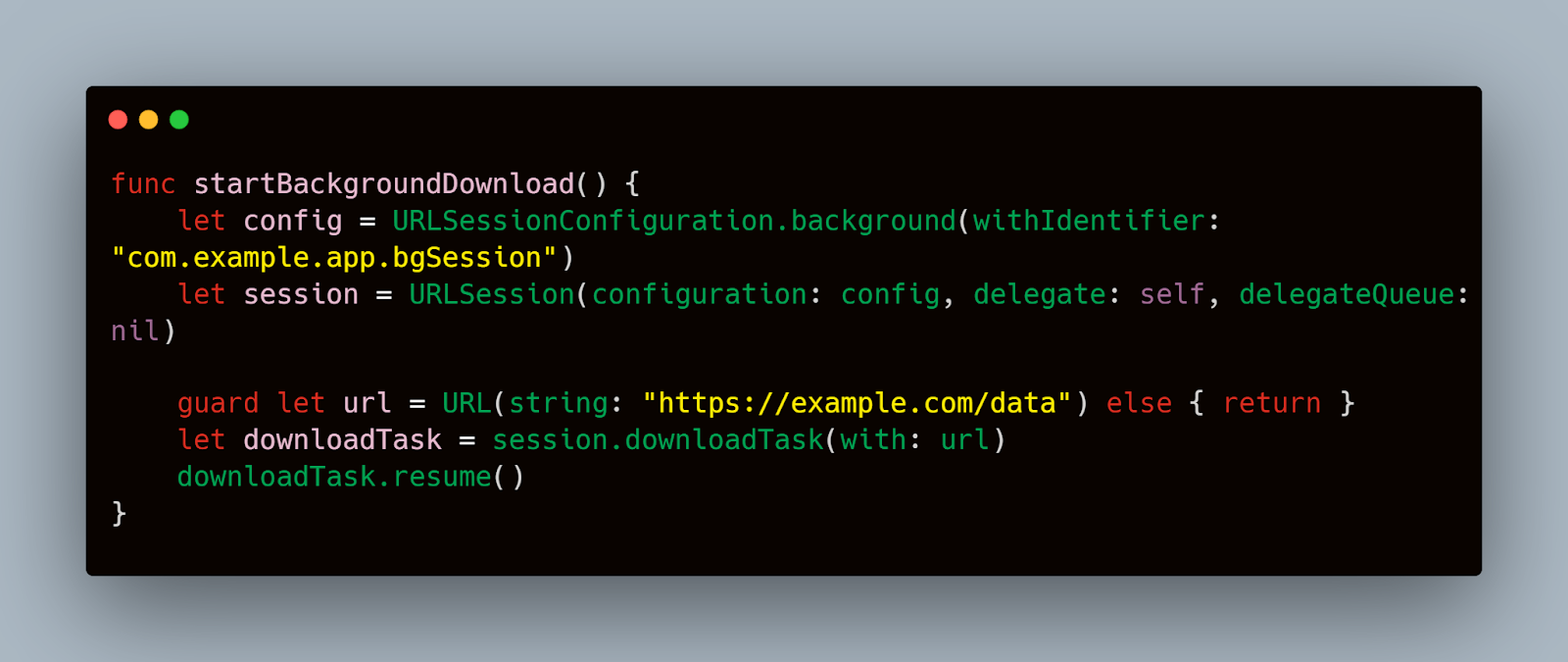
This code snippet sets up a background download task that continues even if the app goes into the background.
- Handling Completion of Background Tasks: Ensure that the completion handler of your network tasks manages the data correctly and updates the app’s state as needed. This is crucial for tasks that must process the fetched data immediately or prepare it for when the app returns to the foreground.

Testing the Reliability of Network Tasks in Background
To test the reliability and effectiveness of background network tasks, simulate network conditions and app backgrounding in your test suite:
- Simulating Network Conditions: Use tools like the Network Link Conditioner on iOS to simulate various network environments—such as low bandwidth or high latency—and observe how your background tasks cope.
- Automated Testing of Background Tasks: Automate the testing process by using XCTest to simulate background conditions and verify the behavior of network requests.
Swift
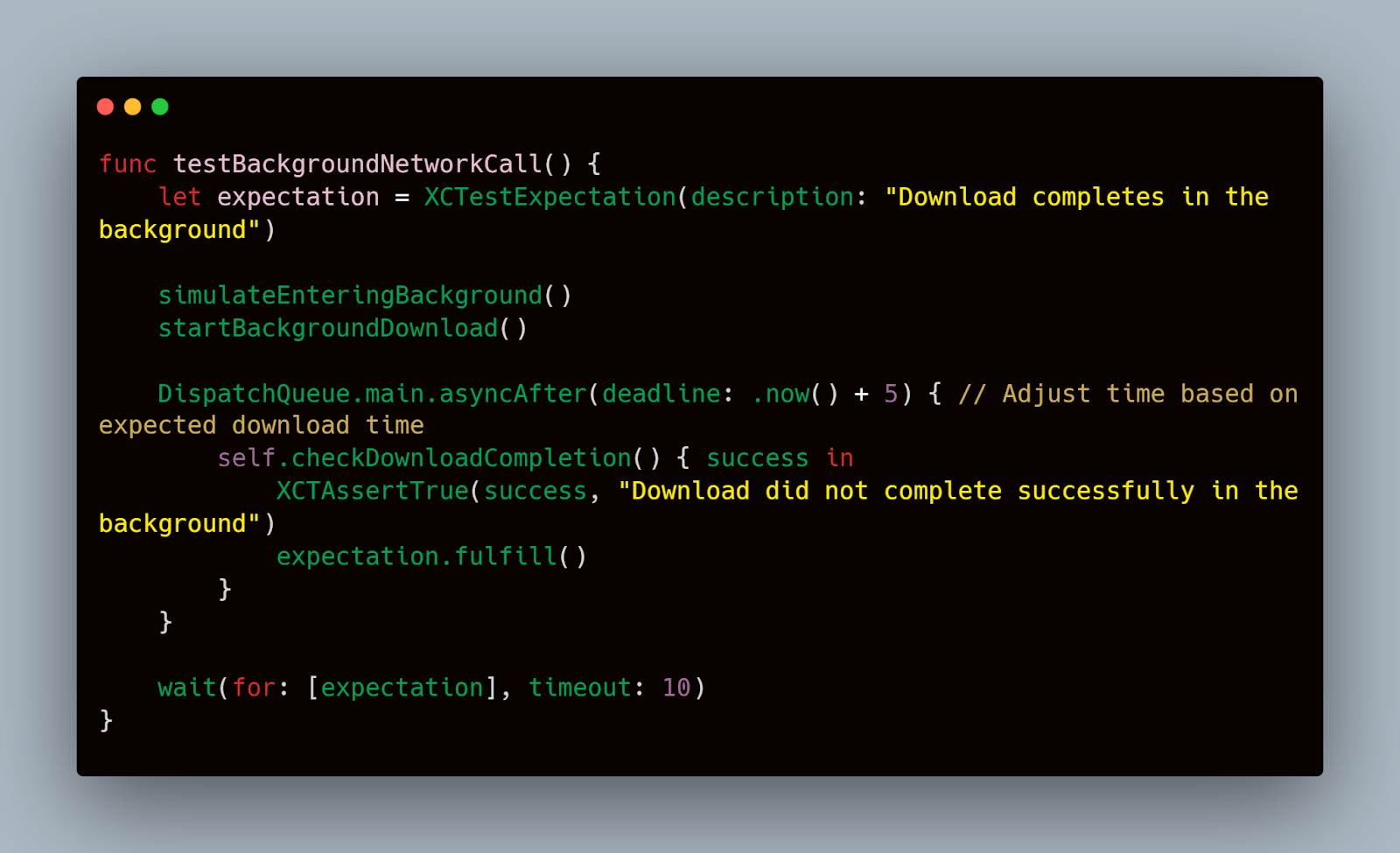
This test checks whether a background download completes as expected under simulated background conditions.
Monitoring App Behavior in Background Mode
When an iOS application enters background mode, it's critical to ensure it continues to operate efficiently without consuming unnecessary resources.
Tools for Monitoring Background Performance
Instruments in Xcode is a powerful toolset that can be used to monitor various aspects of app performance, including memory usage, CPU load, and network activity. Here’s how you can leverage Instruments to observe and optimize your app’s background behavior:
- Activity Monitor: This instrument allows you to track the overall resource usage of your app. It is particularly useful for identifying spikes in CPU or memory usage that could indicate inefficient background processing.
- Leaks: The Leaks instrument helps you detect memory leaks in your application, which can often occur during transitions between foreground and background states if resources are not managed correctly.
- Network: Use the Network instrument to monitor any network activity that your app performs while in the background. This is crucial for ensuring that your app adheres to Apple’s guidelines for background network use.
Here's a simple guide on setting up Instruments for background monitoring:
- Launch Xcode and go to your project.
- Select your app’s target and device for profiling.
- Choose Product > Profile from the top menu to open Instruments.
- Select the instruments you need (e.g., Activity Monitor, Leaks, Network) and start recording while you simulate background mode in your app.
Implementing Code to Facilitate Monitoring
In addition to using external tools, implementing diagnostic code within your app can help track down issues more directly. For instance, logging key events and states can provide insights when the app moves to the background:
Swift
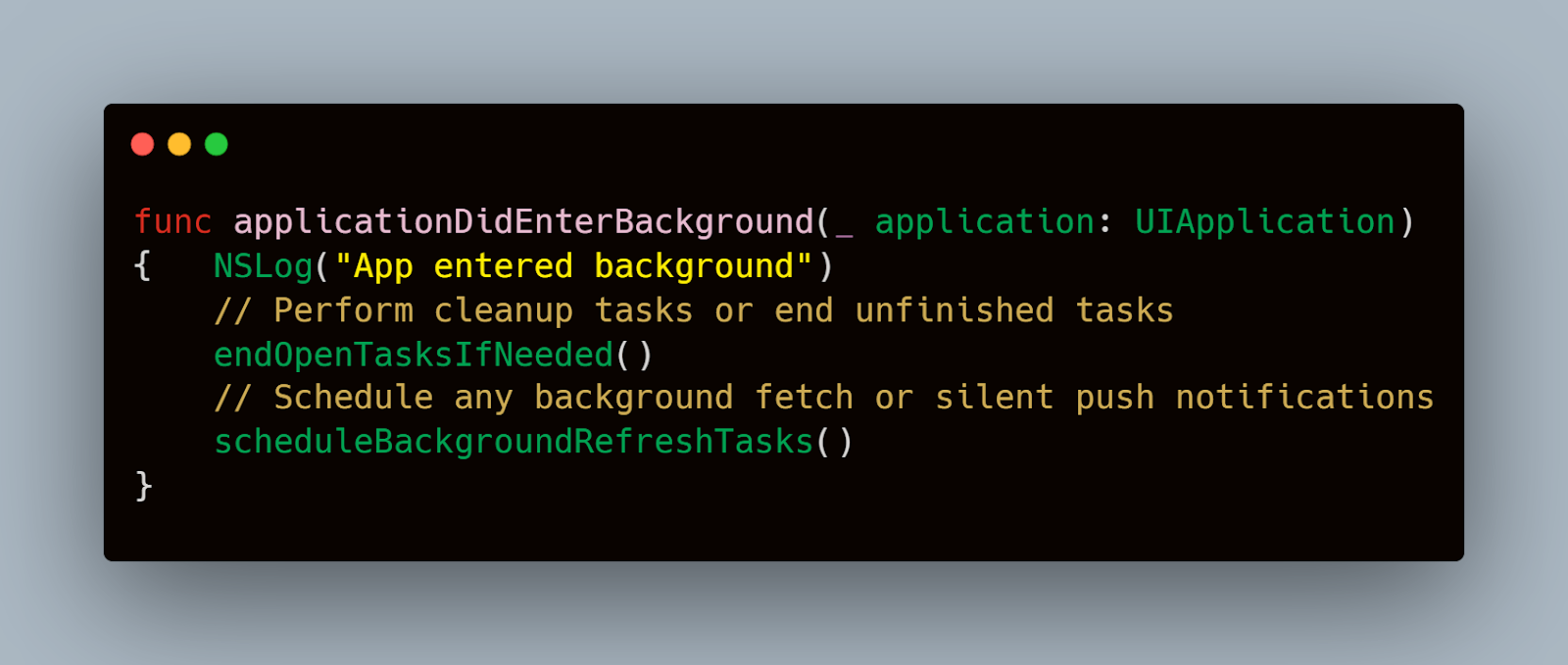
This implementation helps in understanding the app’s state transitions and assists in debugging issues that might only occur in the background.
Analyzing Data for Optimization
Once you collect data through Instruments and internal logs, analyze it to identify areas where the app's performance can be improved. Look for patterns such as unnecessary data fetching, excessive memory use, or prolonged network connections that could be optimized.
While manual monitoring is effective, incorporating automated testing can provide continuous feedback without the need for constant supervision. For example, using a service like Mobot to perform routine background checks can simulate real-world usage patterns across different devices and network conditions. This not only saves time but also ensures a thorough examination of the app’s behavior in background scenarios.
Integration with Third-Party Services
Integrating third-party services into an iOS app is common practice, enhancing functionality without the need for internal development. However, managing these integrations when the app transitions to and operates in the background can be challenging.
Challenges of Third-Party Integrations in Background Mode
Third-party services, such as location tracking, data syncing, and messaging platforms, often require continuous operation, even when the app is not in the foreground. Ensuring these services function seamlessly can impact user satisfaction and app reliability. Common challenges include:
- Maintaining live connections: Services that rely on persistent connections may face interruptions when the app backgrounds, unless properly managed.
- Handling data consistency: Syncing data between the app and third-party services must be resilient to changes in app state to prevent data loss or corruption.
- Energy and resource management: Minimizing the battery and data consumption of third-party services is crucial, as excessive use can lead to poor user experiences.
Best Practices for Integration
- Implementing Background Tasks: For services that need to run in the background (like audio streaming or GPS), use specific APIs provided by iOS. For example, background fetch or silent push notifications can be used to periodically refresh data:
Swift

2. Testing Service Continuity: Use automated tests to simulate background conditions and verify that third-party services resume or sustain functionality as expected:
Swift

- Monitoring and Optimization: Regularly monitor the performance of third-party services using Instruments. Look for unexpected resource usage and optimize accordingly.
While the XCTest framework provides a solid foundation for testing, leveraging a platform like Mobot for more complex scenarios can enhance your testing strategy. Mobot can simulate real-world usage conditions across multiple device configurations, which is ideal for thorough testing of third-party integrations in background modes.
Using Mobot, you can automate interactions that trigger background processes, check for service availability continuously, and measure the impact on system resources, providing a more comprehensive understanding of how well third-party services integrate with your app’s lifecycle.
Edge Case Scenarios and Exception Handling
Testing edge case scenarios and implementing strong exception handling are critical to ensuring that your iOS app operates reliably, especially when it transitions between foreground and background states.
Identifying Edge Case Scenarios
Edge cases in backgrounding might include scenarios where network connections drop as the app goes into the background, or where the app receives a burst of notifications that it must process while not actively in use. Identifying these cases involves understanding the limits and constraints of iOS background execution and how your app interacts with system resources:
- Low Memory Warnings: Test how your app behaves when iOS purges background apps due to low memory.
- Interrupted Tasks: Simulate interruptions, like incoming calls or system updates, during critical background operations.
- Rapid State Changes: Check the app’s resilience when it rapidly switches between background and foreground states.
Testing Strategies
To effectively test these edge cases, automate scenarios using a combination of unit tests and integration tests. XCTest framework in iOS provides tools for simulating background conditions:
Swift
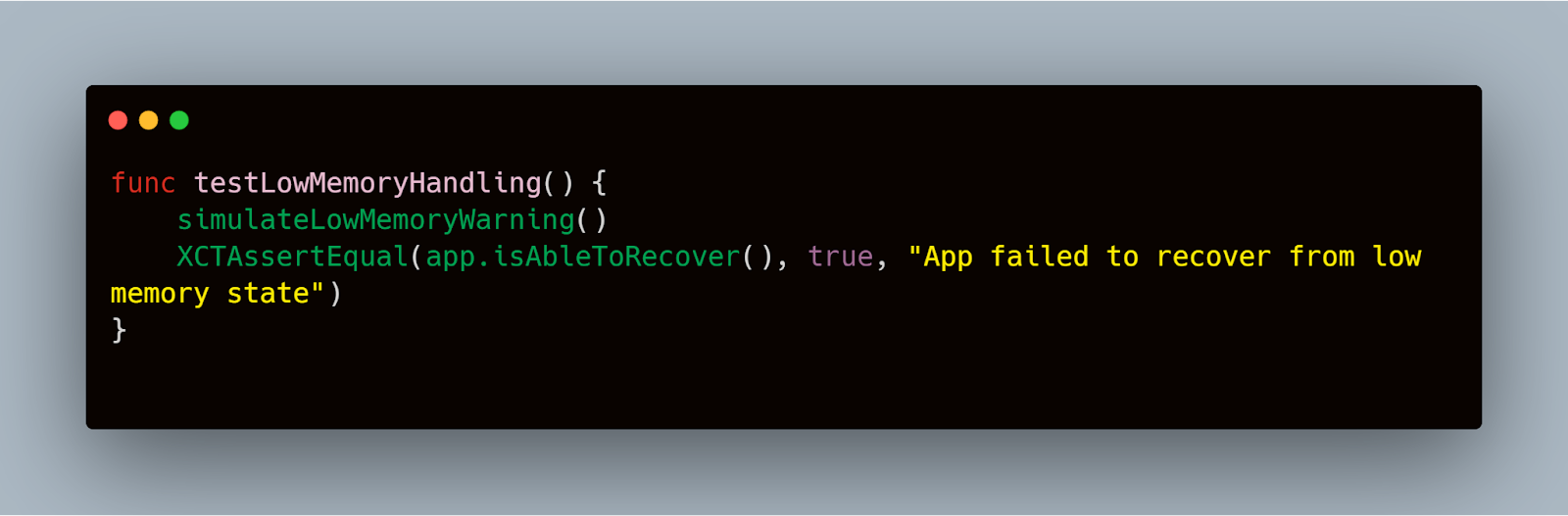
In this example, simulateLowMemoryWarning() would be a method implemented to trigger the iOS low memory warning, and isAbleToRecover() checks if the app can restore its state appropriately.
Exception Handling Best Practices
Proper exception handling in background modes is crucial. Implement try-catch blocks around code that might fail and ensure that the app can recover gracefully:
Swift

Use logs to provide insights into background operations, aiding in debugging and further optimization:
Swift

While XCTest allows for thorough testing of background scenarios, incorporating tools like Mobot can elevate the testing process. Mobot can automate complex user behaviors and simulate real-world conditions, including network variability and interaction patterns that might trigger edge cases.
Automate and Test App Backgrounding on iOS with Mobot
Mobot streamlines the automation and testing of app backgrounding on iOS, ensuring your applications handle state transitions seamlessly and maintain optimal performance. Mobot can simulate scenarios where apps move to the background and resume, validating state persistence, resource management, and the proper execution of background tasks. It supports a wide range of iOS devices and versions, enabling comprehensive testing across different environments. Mobot's automation capabilities reduce manual testing efforts, while its robust reporting and real-time monitoring provide actionable insights into app behavior during backgrounding. Additionally, seamless integration into CI/CD pipelines ensures continuous quality assurance, helping enterprises deliver reliable and high-performing mobile applications with confidence.
Conclusion
Successfully testing backgrounding behavior in iOS apps is crucial for ensuring that applications perform reliably and efficiently, regardless of the user’s direct engagement with the app interface. It covered critical aspects of setting up a comprehensive test environment, automating background state transitions, handling network calls, and monitoring app behavior in background mode. It also touched on the integration of third-party services and the importance of managing edge cases and exceptions effectively. By implementing these strategies and leveraging tools like Mobot for automated testing, developers and QA teams can achieve thorough coverage and more predictable app behavior, enhancing user satisfaction and trust in your application. As iOS continues to evolve, maintaining a rigorous approach to background testing remains paramount, presenting new challenges and opportunities for optimizing app performance in the background.