Explore effective strategies for Bluetooth testing on iOS, ensuring your apps perform seamlessly and meet user expectations.

Introduction
OS devices are widely used in many environments, from personal to professional settings, making them a prime platform for Bluetooth-enabled applications. Testing Bluetooth functionality on these devices is critical because it ensures that your application provides a reliable and consistent user experience. Bluetooth testing can verify that your app maintains stable connections, handles data correctly, and interacts seamlessly with Bluetooth-enabled devices.
Common Challenges in Bluetooth Testing
Testing Bluetooth functionality on iOS presents several unique challenges:
- Device and OS Fragmentation: Different iOS devices and versions can exhibit varying behaviors in how they handle Bluetooth. Ensuring compatibility across all these variations is vital.
- Environment Variability: Bluetooth performance can be significantly affected by physical obstructions, signal interference, and the presence of multiple devices. Replicating these conditions in a test environment can be challenging.
- Security and Privacy Issues: With increasing concerns about data security, testing for potential vulnerabilities in Bluetooth implementations is critical.
- User Experience Consistency: From pairing processes to maintaining connections, the user's experience should be smooth and intuitive across all device types and iOS versions.
Understanding Bluetooth Protocols and iOS Support
When starting on Bluetooth testing for iOS devices, it's crucial to have a solid understanding of the Bluetooth protocols supported by iOS and how the system manages Bluetooth communications. This knowledge forms the backbone of effective testing strategies and ensures that applications perform optimally across all supported devices.
Overview of Bluetooth Protocols Supported by iOS
iOS supports a range of Bluetooth protocols, each designed to cater to different types of applications. The most commonly implemented protocols include:
- Classic Bluetooth (BR/EDR): Used primarily for streaming audio and basic data transfer.
- Bluetooth Low Energy (BLE): Optimized for applications requiring periodic or intermittent data transfer with minimal energy consumption.
- Bluetooth 5.0 and beyond: Offers advancements in range, speed, and broadcasting capabilities, enhancing device interconnectivity.
Developers and testers must ensure their applications are compatible with these protocols to leverage the full capabilities of iOS devices. For instance, an app designed for a fitness tracker would largely rely on BLE to maintain prolonged connectivity without draining the device’s battery.
How iOS Handles Bluetooth Communications
iOS employs a robust framework to manage Bluetooth communications, ensuring security, efficiency, and a seamless user experience. Key components include:
- Core Bluetooth framework: Allows apps to communicate with BLE devices. This framework is crucial for apps that need to interact with health devices, home automation systems, and more.
- External Accessory framework: Supports communication with Bluetooth-enabled hardware accessories that are part of the MFi Program.
Code Snippet: Scanning for BLE Devices on iOS
Here’s a basic example of how an iOS application can scan for BLE devices using Swift:
swift
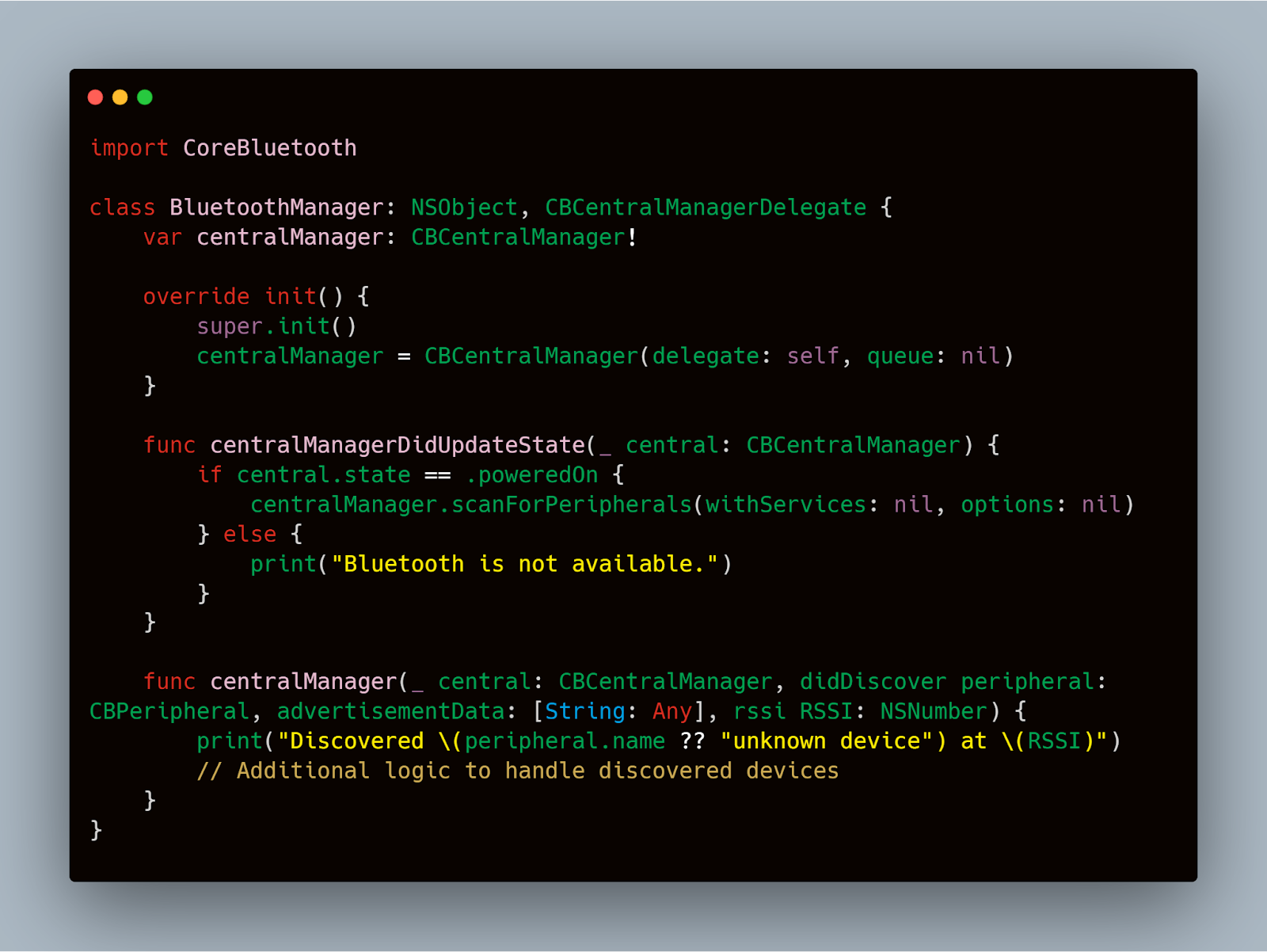
This snippet highlights the initialization and use of the CBCentralManager to scan for nearby BLE devices, demonstrating the application of the Core Bluetooth framework.
Understanding the intricacies of these frameworks and how they interact with different Bluetooth protocols is essential for crafting comprehensive test cases. This ensures that your application behaves as expected under various scenarios and aligns with the stringent requirements of iOS systems.
Setting Up the Testing Environment
Creating an effective testing environment is crucial for the accurate assessment of Bluetooth functionality on iOS devices. This involves configuring both hardware and software to simulate real-world conditions, which helps in uncovering potential issues before the application is deployed.
Required Hardware and Software
To begin with, you will need a variety of iOS devices with different configurations. This includes devices with varying levels of Bluetooth support—from older models that support Classic Bluetooth to the latest devices supporting Bluetooth 5.0 and beyond. Here’s a checklist for setting up your hardware:
- Multiple iOS Devices: Include a range of devices with different iOS versions and Bluetooth chip versions to cover various user scenarios.
- Bluetooth Peripherals: Collect various Bluetooth accessories like speakers, headphones, wearables, and car systems to test connectivity and performance.
- Network Interference Generators: Tools to simulate different environmental conditions, such as Wi-Fi signals, microwave interference, and other RF noises.
On the software side, ensure you have the latest version of Xcode installed, which includes all necessary tools and simulators for developing and testing iOS applications. Additionally, it's beneficial to have access to the following:
- Bluetooth Scanner Apps: Applications like LightBlue Explorer or nRF Connect to monitor Bluetooth activity and troubleshoot connectivity issues.
- Performance Monitoring Tools: Software to analyze the battery usage, memory leaks, and processor load during Bluetooth operations.
Configuring iOS Devices for Bluetooth Testing
Setting up your iOS devices correctly is key to obtaining reliable test results. Here’s how to configure them:
- Enable Developer Mode: Go to Settings > Privacy & Security > Developer Mode and follow the prompts to enable developer settings.
- Disable Automatic Connections: In the Bluetooth settings, turn off the ‘Auto-Connect’ feature to manually control device connections during testing.
- Update iOS to the Latest Version: Ensure all devices are running the latest iOS version available to test the newest features and security patches.
Example Code: Enabling Bluetooth Logging on iOS
For deeper insights into Bluetooth interactions, enabling system logging can be invaluable. Here’s how you can programmatically enable Bluetooth logging using a simple Swift script:
Swift

This script initializes logging for Bluetooth-related activities, helping you capture detailed information during test sessions.
Utilizing Mobot for Repetitive Testing
While much of Bluetooth testing requires manual interaction, repetitive tasks can be automated using tools like Mobot. This robotic solution can automate physical interactions with devices, such as turning Bluetooth on and off, connecting to different peripherals, and switching between apps, which can significantly enhance testing efficiency and accuracy.
Automated Testing Tools
Automating Bluetooth tests on iOS can significantly streamline the testing process, reduce manual errors, and ensure consistent testing outcomes across different devices and scenarios.
Core Tools for Automated Bluetooth Testing
- XCTest Framework: XCTest provides a robust suite of functionalities for unit and UI testing of iOS applications. For Bluetooth, XCTest can be used to automate the testing of Bluetooth-enabled app features by simulating interactions with Bluetooth devices.
- Bluetooth Test Server (BTS): BTS is a simulated environment that allows you to create virtual Bluetooth devices. These devices can interact with your app as real devices would, enabling you to test various scenarios without the need for physical hardware.
- CI/CD Integration: Integrating Bluetooth tests into a Continuous Integration/Continuous Deployment pipeline ensures that Bluetooth functionality is consistently tested at every stage of the development process. Tools like Jenkins, CircleCI, and GitHub Actions can be configured to run automated Bluetooth tests each time code is pushed to a repository.
Implementing XCTest for Bluetooth Interaction
Using XCTest for Bluetooth involves creating test cases that can simulate user interactions and Bluetooth connectivity scenarios. Below is an example of how you might write a test case to check if your application correctly discovers and connects to Bluetooth devices:
Swift

This test initializes a CBCentralManager object, starts scanning for peripherals, and checks if Bluetooth is enabled on the device, all within an automated test environment.
Leveraging Mobot for Repetitive Interaction Tests
While software-based tools are crucial, Mobot provides a unique advantage for physical interaction testing. This includes automating tasks such as switching Bluetooth on and off on an actual device, connecting to various peripherals, and testing the physical range and barriers in Bluetooth connectivity. Incorporating Mobot in automated tests can help simulate and test user interactions more realistically than software simulations alone.
Manual Testing Techniques
While automation plays a critical role in testing, manual testing remains indispensable, especially when assessing user experience and interaction nuances with Bluetooth on iOS devices.
Step-by-Step Guide to Manual Bluetooth Testing
Manual testing involves a series of steps that simulate real-world usage, ensuring that your application interacts correctly with Bluetooth devices under various scenarios. Here’s a structured approach:
- Initial Pairing and Connection Setup
- Test the initial discovery and pairing process with a range of Bluetooth devices (e.g., headsets, wearables, car systems).
- Verify that the application handles the pairing process smoothly and manages any user prompts or errors effectively.
- Reconnection Logic
- Disconnect and reconnect devices multiple times to test the stability and reliability of reconnections.
- Check how the application responds to interruptions, such as incoming calls or switching between different Bluetooth devices.
- Cross-Device Compatibility
- Pair with devices from various manufacturers and with different Bluetooth versions to assess compatibility.
- Identify any device-specific issues or inconsistencies in connectivity and functionality.
- Data Transfer and Management
- Test data syncing capabilities, if applicable, such as transferring files or streaming audio.
- Evaluate the performance and integrity of data being transferred, looking for any corruption or loss of data.
- User Interface and Notifications
- Ensure that all user interface elements related to Bluetooth functionality (e.g., status indicators, error messages) are clear and function as expected.
- Test notifications and alerts related to Bluetooth events to verify their accuracy and timeliness.
Common Manual Testing Scenarios
- Interference Testing: Simulate environments with high interference (other Bluetooth devices, Wi-Fi signals, microwave ovens) to test how well the application maintains Bluetooth connectivity.
- Range Testing: Move the connected device progressively farther from the iOS device to test the range limits and how the application notifies the user of signal degradation or loss.
- Battery Drain Analysis: Monitor the battery consumption of the iOS device while connected to Bluetooth, especially during extended use cases like streaming music or during active workout sessions.
Tips for Effective Manual Testing
- Document Everything: Keep detailed logs of all tests, including device configurations, steps taken, and any issues encountered. This documentation is invaluable for troubleshooting and for future regression testing.
- User Scenario Simulation: Engage in role-playing different user scenarios to cover a variety of real-life uses, such as using the app in a busy office, at home, or in a vehicle.
- Feedback Loop: Incorporate feedback from real users into the manual testing process. User insights can reveal overlooked issues and provide new perspectives on the app’s Bluetooth functionality.
Debugging Common Bluetooth Issues on iOS
Debugging is a critical phase in the Bluetooth testing process on iOS, as it helps identify and resolve issues that could impair user experience.
Tools and Strategies for Troubleshooting
To effectively diagnose and fix Bluetooth issues on iOS, it's essential to use a combination of system logs, third-party tools, and custom debugging techniques:
- Console App for System Logs: The Console app on macOS can be connected to an iOS device to access system logs in real time. These logs can provide invaluable insights into Bluetooth events and errors.
- Bluetooth Diagnostic Utilities: Tools like Apple's Bluetooth Explorer and PacketLogger, part of the Hardware IO Tools for Xcode, allow deeper inspection of Bluetooth communication and packet transfer, which is crucial for debugging complex issues.
- Custom Debugging Code: Implementing additional logging within your application can help trace Bluetooth operations and identify where failures occur. For instance, adding detailed logs around connection points and data transfer sections can pinpoint exact failure moments.
Case Studies of Typical Bluetooth Bugs and Their Resolution
Case Study 1: Connection Instability
- Problem: Users report frequent disconnections with Bluetooth headphones during calls.
- Diagnostic Approach: Utilize system logs to monitor disconnections and check for any common patterns or error messages.
- Solution: Updating the application to handle Bluetooth audio routing more efficiently and implementing a more robust reconnection strategy.
Case Study 2: Data Transfer Inconsistency
- Problem: Inconsistent data transfer rates observed when syncing data from a fitness band to the iOS app.
- Diagnostic Tools: Use PacketLogger to analyze the data packets being transferred and identify any anomalies or interruptions.
- Solution: Refactor the data handling code to include retries and checksum validations to ensure data integrity.
Example Code: Adding Debugging Logs to iOS Bluetooth App
Here’s a simple example of how you might add debugging logs to a Swift function that handles Bluetooth connectivity:
Swift
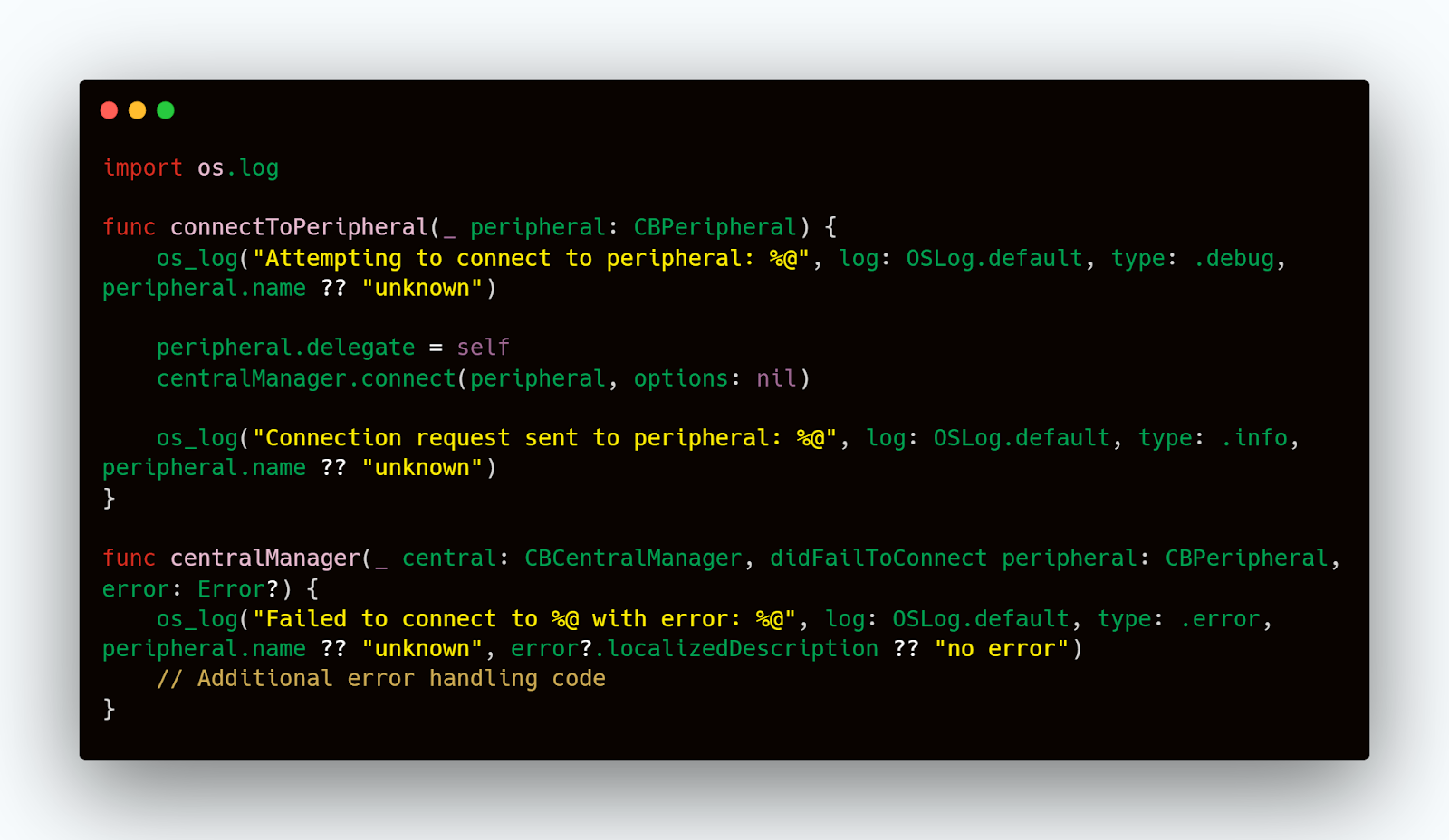
This code snippet demonstrates how to implement logging for connecting to a Bluetooth peripheral, providing clear insights into the connection process and potential points of failure.
Conclusion
Successfully testing Bluetooth functionality on iOS devices demands a blend of rigorous testing methods, advanced tools, and a keen focus on user experience. This guide has outlined the importance of integrating both automated and manual testing strategies, utilizing powerful diagnostic tools, and adopting a user-centric approach to ensure the application's functionality is both intuitive and reliable. Staying current with technological advances and updates in iOS and Bluetooth technologies is crucial for maintaining the efficacy of your testing strategies. By applying the insights and techniques discussed, developers and testers can confidently enhance the quality of their applications, ensuring seamless Bluetooth interactions that meet the high expectations of their users.